Redux is too complicated right ? so, let’s try a new react global state management called Jotai. Find out how it work, how to use it ? And in the last section of the blog, I’ll show you guys the usecase for redux and jotai
1. What’s Jotai ?
Jotai is a lightweight state management library specifically designed for React applications. With Jotai, you can easily manage and share state across components without the need for complex setup or boilerplate code. It embraces a simple and intuitive approach, allowing you to define state atoms and derive derived state atoms. Jotai promotes a declarative and composable programming style, making it a powerful tool for handling state in your React projects.
2. How it works ?
Jotai simplifies state management in React applications by using an atom-based approach. Atoms are units of state that can be created and accessed throughout your application.
- Here’s a simplified overview of how Jotai works:
- Define Atoms: You create atoms using the
atom
function. An atom represents a piece of state that can be read and updated. - Use Atoms: In your components, you can use the
useAtom
hook to read and update the value of an atom. When an atom’s value changes, any component that uses that atom will automatically re-render. - Derive Derived Atoms: Derived atoms can be created by using the
deriveAtom
function. Derived atoms depend on one or more atoms and are automatically updated whenever their dependencies change. - Update Atoms: To update the value of an atom, you can use the
setAtom
function provided by theuseAtom
hook. This triggers a re-render of components that use the atom. - Optimize Re-renders: Jotai uses an optimized rendering mechanism, which means that only the components that depend on the changed atoms will re-render, improving performance.
- Compose Atoms: You can compose atoms to build more complex state structures. This allows you to encapsulate related state and make your code more modular and reusable.
- Quick review how we use jodai
3. Let’s get start
- Create a new react app using vite
yarn create vite
- Install Jotai
yarn add jotai
- Create some demo components
//src/components/Home.tsx
const Home = () => {
return (
<>
<h1>Home</h1>
</>
);
};
export default Home;
//src/components/User.tsx
const User = () => {
return (
<>
<h1>User</h1>
</>
);
};
export default User;
- Import it in App.tsx
import "./App.css";
import User from "./components/User";
import Home from "./components/Home";
function App() {
return (
<>
<User />
<Home />
</>
);
}
export default App;
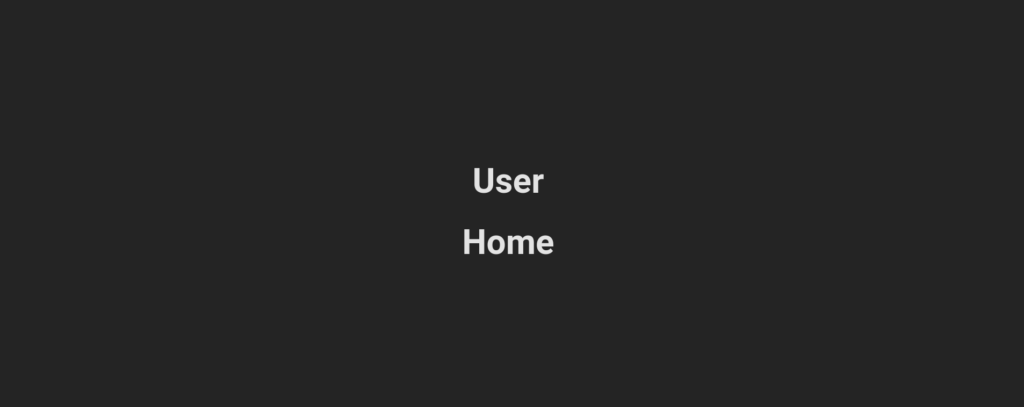
- Simple right ?, now magic’s time. Let’s add atom to use jotai in App.tsx, just for demo you can create a direactory and export all atoms
import "./App.css";
import { atom } from "jotai";
import User from "./components/User";
import Home from "./components/Home";
export const lightModeAtom = atom("dark");
function App() {
return (
<>
<User />
<Home />
</>
);
}
export default App;
- Here, we create an atom call lightModeAtom, you can imagine atom is a global state that can use in all components of your react app. And the lightMode state have a defaut value is light
- Let’s try to get this state’s value in our User‘s component
import { useAtom } from "jotai";
import { lightModeAtom } from "../App";
const User = () => {
const [lightMode, setLightMode] = useAtom(lightModeAtom);
return (
<>
<h1>User</h1>
<p>Current lightmode: {lightMode}</p>
</>
);
};
export default User;
- Look simple right, just like we all use the useState hook everyday. Now we use useAtom hook and pass in the atom that we’ve created in the App.tsx file. Let see how it works
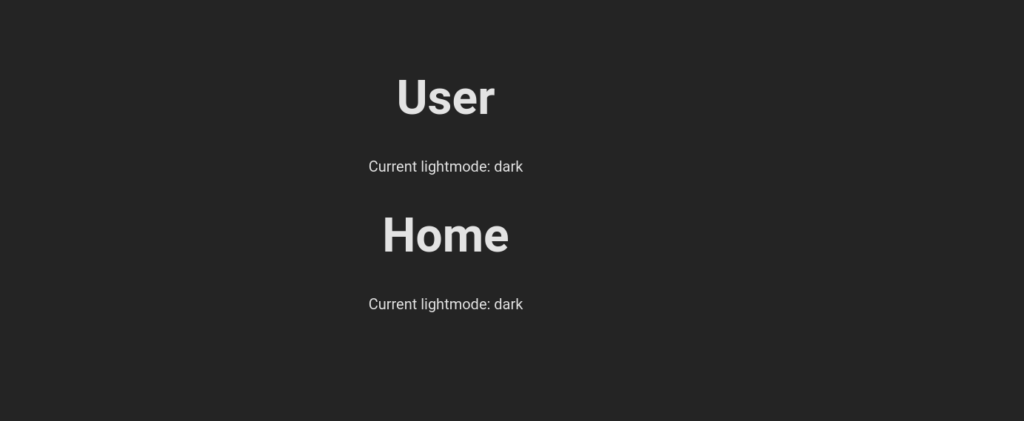
- Now we can access the lightMode state in both Home and User component. Now let’s try to update the state, use the set function returned from the useAtom hook
const Home = () => {
const [lightMode, setLightMode] = useAtom(lightModeAtom);
const toggelMode = () => {
setLightMode(lightMode === "dark" ? "light" : "dark");
}
return (
<>
<h1>Home</h1>
<p>Current lightmode: {lightMode}</p>
<button onClick={toggelMode}>toglle mode</button>
</>
);
};
- Here is the result:
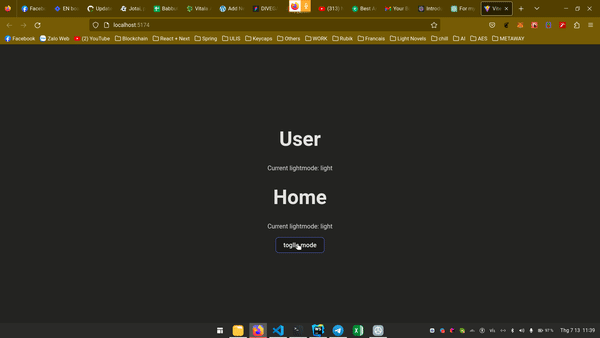
- It works like a charm right ?
- It’s just a quick demo of Jodai, this library have some more useful functions. Vist the docs for more: https://jotai.org/
4. Can jotai repalce redux ?
Quick answer is NO ! In fact, we should use both base on the state that you want to store. Jotai is just a simple state management to store some piece of state and decrease the re-render by assume that only component depennds on atom will be re-render when the atom udpate. Imagine when you have a small state that you need to use in a lot of component ex: light or dark mode, a open state for a global modal,… Setup redux for a small state like this is painfull and unecessary. We can use the react context but it may cause too many re-render then jotai is the number one solution.
With more complex state for more complex project we still should use redux because:
- Ecosystem and Tooling: Redux has a larger ecosystem and tooling support, including a wide range of middleware, dev tools, and integration with other libraries. Jotai, being a newer library, may have a smaller ecosystem and fewer community-contributed packages.
- Time-Travel Debugging: Redux offers powerful time-travel debugging capabilities through tools like Redux DevTools. This allows you to replay and inspect the state changes over time, which can be extremely useful for debugging complex applications. Jotai does not have built-in support for time-travel debugging.
- Middleware: Redux provides a middleware system that allows you to intercept and modify actions and state updates. This can be useful for handling side effects, asynchronous operations, or applying additional logic before state changes. Jotai does not have a built-in middleware system, so you would need to handle side effects and complex state updates through other means.
- Learning Curve: Redux has been around for a longer time and has established patterns and conventions. This means that there is a wealth of documentation, tutorials, and community resources available to help you learn and use Redux effectively. Jotai, being a newer library, might have a smaller learning resource base in comparison.
In summary, consider using Jotai for smaller projects or when simplicity and ease of integration are your priorities. If you anticipate your application growing in size and complexity, or if you need advanced features and broad ecosystem compatibility, Redux might be the more suitable choice. Ultimately, both libraries can effectively handle state management in React, so evaluate your specific requirements and choose the one that aligns best with your needs.
It’s CodingCat again thanks for reading <3. Stay tune for more
_CodingCat 2023_